包阅导读总结
1.
关键词:gRPC、Retry Policies、Java Applications、Distributed Systems、Transient Failures
2.
总结:
本文探讨了在 Java 应用中为 gRPC 请求配置重试策略,介绍了重试策略的重要性、关键参数,通过示例详细展示了服务配置、服务实现、服务器与客户端的创建,以及如何测试重试策略,最后总结了实现和配置的过程。
3.
主要内容:
– gRPC 简介
– 是高性能 RPC 框架,用于微服务间高效通信,网络请求可能因多种原因失败。
– 重试策略的重要性
– 定义 gRPC 客户端在请求失败时的行为,处理分布式系统中的临时问题。
– gRPC 重试策略
– 支持在服务配置中定义,可指定多种参数,如最大尝试次数、初始和最大重试延迟等。
– 配置 gRPC 重试策略
– 定义服务配置,创建包含重试策略配置的 JSON 文件。
– 实现 gRPC 服务,包括定义 proto 文件、创建服务实现类、创建服务器。
– 实现 gRPC 客户端,应用服务配置。
– 测试重试策略,通过随机模拟失败来检验策略效果。
– 结论
– 总结了在 Java 应用中为 gRPC 请求配置重试策略的过程。
思维导图:
文章地址:https://www.javacodegeeks.com/configuring-grpc-retry-policies-in-java-applications.html
文章来源:javacodegeeks.com
作者:Omozegie Aziegbe
发布时间:2024/8/7 15:14
语言:英文
总字数:1261字
预计阅读时间:6分钟
评分:84分
标签:grpc,重试策略,Java,微服务,故障容忍
以下为原文内容
本内容来源于用户推荐转载,旨在分享知识与观点,如有侵权请联系删除 联系邮箱 media@ilingban.com
gRPC is a high-performance RPC framework that enables efficient communication between microservices. However, network requests can fail due to various reasons like network congestion, server overload, or temporary unavailability. To handle such failures, gRPC provides a retry mechanism that allows clients to automatically retry failed requests. In this article, we will explore how to configure a retry policy for gRPC requests.
1. Why Retry Policies?
A retry policy defines how a gRPC client should behave when a request fails. They are essential for dealing with temporary issues in distributed systems. These issues, like network glitches, temporary service outages, or brief overloads, often fix themselves. A retry policy can handle these problems automatically, helping our application recover smoothly and continue functioning correctly.
1.1 gRPC Retry Policies
gRPC supports retry policies defined in the service configuration. These policies can be specified in a JSON or YAML format and include various parameters such as the maximum number of attempts, the initial and maximum retry delays, and back-off strategies. Here is a tabular representation of the key components of gRPC retry policies:
Parameter | Description | Example Value |
---|---|---|
Max Attempts | The maximum number of retry attempts. | 5 |
Initial Backoff | The initial delay before the first retry attempt. | 0.1s (100 milliseconds) |
Max Backoff | The maximum delay between retry attempts. | 1s (1 second) |
Backoff Multiplier | A multiplier applied to the backoff interval after each retry. | 2 |
Retryable Status Codes | The status codes that will trigger a retry. | UNAVAILABLE , DEADLINE_EXCEEDED |
Jitter | A random amount of time added to the backoff to prevent thundering herd problem (not always used). | 0.2s |
Timeout | The maximum amount of time to wait for a response before retrying. | 2s |
2. Configuring Retry Policies in gRPC
To configure retry policies in gRPC, we need to define a service configuration and apply it to our client. Let’s look at an example scenario where we have a gRPC service called UserService
with a method GetUser
that fetches user details. We will configure a retry policy for this method to handle transient failures.
2.1 Define the Service Configuration
Create a JSON file named service_config.json
in your resources directory. This file will contain the retry policy configuration.
{ "methodConfig": [ { "name": [ { "service": "grpcretryexample.UserService", "method": "GetUser" } ], "retryPolicy": { "maxAttempts": 5, "initialBackoff": "0.5s", "maxBackoff": "30s", "backoffMultiplier": 2, "retryableStatusCodes": [ "UNAVAILABLE", "DEADLINE_EXCEEDED" ] } } ]}
service
: Specifies the service name (UserService
).method
: Specifies the method name (GetUser
).maxAttempts
: Maximum number of retry attempts (5 in this case).initialBackoff
: Initial delay before the first retry attempt (0.5 seconds).maxBackoff
: Maximum delay between retry attempts (30 seconds).backoffMultiplier
: Multiplier for the backoff interval (2).retryableStatusCodes
: Status codes that trigger a retry (UNAVAILABLE
andDEADLINE_EXCEEDED
).
2.2 Implement the gRPC Service
First, let’s define the proto file (user_service.proto
) for our UserService
.
syntax = "proto3";option java_multiple_files = true;option java_package = "com.jcg.grpc";package grpcretryexample;service UserService { rpc GetUser(GetUserRequest) returns (GetUserResponse);}message GetUserRequest { string user_id = 1;}message GetUserResponse { string user_id = 1; string name = 2; string email = 3;}
Here’s an overview of the Proto File:
- Service Definition: The
UserService
service is defined with a single RPC methodGetUser
. - GetUser Method: This method takes a
GetUserRequest
message and returns aGetUserResponse
message. - GetUserRequest: This message contains a single field
user_id
, which is a string representing the user’s ID. - GetUserResponse: This message contains three fields:
user_id
,name
, andemail
, representing the user’s ID, name, and email address, respectively.
The proto file serves as the contract between the client and the server, defining the structure of requests and responses. In this case, the GetUser
method is used to fetch user details based on a provided user ID.
2.3 Implement the gRPC Server
Create a class to implement the UserService
.
import io.grpc.Server;import io.grpc.ServerBuilder;import io.grpc.stub.StreamObserver;import java.io.IOException;import java.util.Random;public class UserServiceImpl extends UserServiceGrpc.UserServiceImplBase { private final Random random = new Random(); @Override public void getUser(GetUserRequest request, StreamObserver<GetUserResponse> responseObserver) { // Simulate a 90% chance of transient failure if (random.nextInt(100) < 90) { responseObserver.onError(io.grpc.Status.UNAVAILABLE .withDescription("Service unavailable") .asRuntimeException()); System.out.println("Service temporarily unavailable; will retry if the policy allows."); } else { GetUserResponse response = GetUserResponse.newBuilder() .setUserId(request.getUserId()) .setName("Allan Gee") .setEmail("allan.geee@jcg.com") .build(); responseObserver.onNext(response); responseObserver.onCompleted(); } } public static void main(String[] args) throws IOException, InterruptedException { Server server = ServerBuilder.forPort(50051) .addService(new UserServiceImpl()) .build() .start(); System.out.println("Server started on port 50051"); server.awaitTermination(); }}
The above UserServiceImpl
class implements the UserServiceGrpc.UserServiceImplBase
abstract class, which is generated from the user_service.proto
file. Here is a breakdown of the code:
- Random Failure Simulation:
- A
Random
object is used to introduce a chance of failure. - The
getUser
method checks the result ofrandom.nextInt(100) < 90
. If it istrue
, the method simulates a transient failure by callingresponseObserver.onError
with anUNAVAILABLE
status. - If it is
false
, it returns a successfulGetUserResponse
containing user details.
- A
- The
main
method sets up a gRPC server on port 50051 and adds theUserServiceImpl
service. The server is started withserver.start()
and will keep running until terminated.
2.4 Implement the gRPC Client
Create a class for the gRPC client that applies the service configuration.
import com.google.gson.Gson;import com.google.gson.stream.JsonReader;import io.grpc.ManagedChannel;import io.grpc.ManagedChannelBuilder;import java.io.*;import java.util.Map;import java.nio.charset.StandardCharsets;public class GrpcClient { public static void main(String[] args) { Gson gson = new Gson(); Map<String, ?> serviceConfig; // Load the service configuration from the JSON file using Gson serviceConfig = gson.fromJson(new JsonReader(new InputStreamReader(GrpcClient.class.getClassLoader() .getResourceAsStream("service_config.json"), StandardCharsets.UTF_8)), Map.class); // Build the channel with retry policy ManagedChannel channel = ManagedChannelBuilder.forAddress("localhost", 50051) .usePlaintext() .disableServiceConfigLookUp() .defaultServiceConfig(serviceConfig) .enableRetry() .build(); UserServiceGrpc.UserServiceBlockingStub stub = UserServiceGrpc.newBlockingStub(channel); GetUserRequest request = GetUserRequest.newBuilder() .setUserId("12345") .build(); try { GetUserResponse response = stub.getUser(request); System.out.println("User: " + response.getName() + ", Email: " + response.getEmail()); } catch (Exception e) { } finally { channel.shutdown(); } }}
In the class above:
- Gson is used to read the
service_config.json
file and convert it to a JSON string. - ManagedChannelBuilder: Creates a channel with the service configuration and enables retries.
- UserServiceGrpc.UserServiceBlockingStub: Creates a blocking stub to call the
GetUser
method. - The client attempts to call the
GetUser
method and prints the user details if successful.
2.5 Testing the Retry Policy
After configuring the retry policy, testing it to ensure it behaves as expected is essential. Note that to simulate transient failures in the gRPC server implementation (UserServiceImpl
), we introduced random failures in the getUser
method.
With this setup, when we run the gRPC client (GrpcClient
), it will encounter transient failures randomly. The client will retry the request according to the specified policy and eventually succeed when the server does not simulate a failure.
Run the Server:
When we run the Server, the output is:
Server started on port 50051
Run the Client:
When we run the client and examine the logs, the server output with the simulated failure (which is highly likely due to the high chance) is:
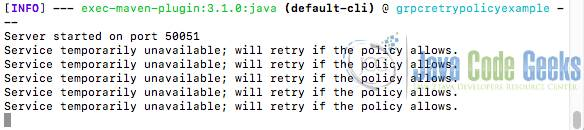
On successful retry when the chance succeeds, we get:
User: Allan Gee, Email: allan.geee@jcg.com
With this setup, our gRPC client will encounter transient failures most of the time, which will trigger the retry mechanism and help test its effectiveness.
3. Conclusion
In this article, we explored how to implement and configure retry policies for gRPC requests in a Java application. We started by defining the service configuration using a JSON file. We then provided a guide on setting up and running both the gRPC server and the client.
4. Download the Source Code
This article explains how to configure a retry policy for gRPC requests in Java.